Understanding Scripts
To help you understand scripts, this section uses an example: a complete script that responds to a specific event. This section also includes information on writing scripts from scratch and a simple tool that can help writing scripts easier.
Topics:
Our First Example
This example is a complete script that responds to an InspectionScheduleAfter event by inserting a new smart notice with information about the scheduled inspection. The example is several lines long, and contains comments at lines 1, 6, 9, 16, 19, and 26 that briefly explain what is happening in each section in the script.
Note that each line that begins with a comment starts with a double slash. The double slash tells Civic Platform to ignore that line. It is good practice to add comments to your scripts.
1 |
|
2 |
|
3 |
|
4 |
|
6 |
|
7 |
|
9 |
|
10 |
|
11 |
|
12 |
|
13 |
|
14 |
|
16 |
|
17 |
|
19 |
|
20 |
|
21 |
|
22 |
|
23 |
|
24 |
|
26 |
|
27 |
|
28 |
|
Another important feature of our first example is that every line that is not a comment line seems to end with a semicolon ( ; ). We can see that lines 2, 3, 4, 7, 10, 11, 12, 13, 14, 17, 24, and 28 end with a semi-colon. The semi-colon tells Civic Platform that it has reached the end of a command, and should execute it. If we look at line 27 we see that it does not end with a semi-colon, but ends with a comma. The comma means that the command continues on to the next line. We see that line 28 ends with a semi-colon. The semi-colon means that there is one command that begins on line 27 and ends on line 28. If we look at lines 20, 21, 22, 23, and 24 we can see that these lines comprise one big command split across five lines to make it easier to read.
If you forget to end your commands with a semi-colon, Civic Platform is forgiving and the script may run correctly, but it is always good practice to end your commands with a semi-colon when necessary. Some kinds of commands do not have to end in a semi-colon. We investigate what kinds of commands end with a semi-colon and what kinds do not in later sections of this document.
Writing And Testing Our First Script
While you are learning to write scripts, it is useful to be able to test simple scripts and see the results immediately without having to attach your script to an event. To do this we use the Script Test page. For information on testing scripts, see Script Testing. When we cover a new sample script in this document you can copy the script and paste it into the Script Text field on the Script Test page. After you have pasted the text of the script into the form, you can click the Submit button to run the script and view the result.
You can also type this script by hand. Typing the script can be a very helpful learning aid when you start with script writing. However, if you make a mistake in typing you receive a message telling you that there is a problem with your script. When you get a message telling you about the problem, check your script to make sure that it matches the example. Another good tip for learning to write script is to try to modify the sample script to see what happens.
Here is our first sample script for testing:
aa.print(‘Hello World.’);
If you have read the earlier sections of this document, you may recognize our sample script. The output of this script is
Hello World.
Let us look at exactly what is happening in our sample script. The script has one line that ends with a semi-colon just like the lines in the first example. The line begins with the two letters ‘aa’. These two letters stand for Civic Platform. This line begins with ‘aa’ because we are going to tell Civic Platform to do something for us. A dot follows the ‘aa’. The dot connects the word ‘aa’ to the word ‘print’, which means that the word ‘print’ is a method of the ‘aa’ object.
An object is a group of associated actions or functions. The previous sample script calls the object aa. The aa object can retrieve data from your database and then use the data to perform tasks. A simple example of the tasks that the aa object is capable of performing is the print task, but there are many tasks that the aa object can perform.
Objects can retrieve information, change stored information, and do many other things for you. Writing and implementing scripts is how we get the aa object (or any other object in JavaScript) to do work with raw data. When we choose a script to initialize in Civic Platform, we are essentially giving a command to our machine. We call the commands we give methods. We learn more about objects and methods in the section Using Objects, Properties, and Methods.
We now know that this line is asking Civic Platform to print something for us. After the word ‘print’ there is a left parenthesis. After the words ‘Hello World.’ there is a right parenthesis. When you are writing scripts, a you must follow a method name by a pair of parentheses. Sometimes there are things in between the parentheses, called parameters. Parameters tell a method how to do its job. When we look at the characters between the parentheses we see a single quote followed by the words Hello World, followed by a period, followed by another single quote. Strings are words, numbers, or punctuation marks that appear between single or double quotes.
We know that this script is telling Civic Platform to print the string ‘Hello World.’, which is exactly what appears in the Script Output box when you use the Script Test page to run this script. For practice, try to change the string passed to the print method of the aa object and see what happens. You can also try to add a second line after the first one with prints out something different, and see what happens then.
Using Jext To Make Writing Scripts Easier
The Jext editor is a freely available text editor with many features that make editing scripts easier. Here is a screenshot of Jext in action:
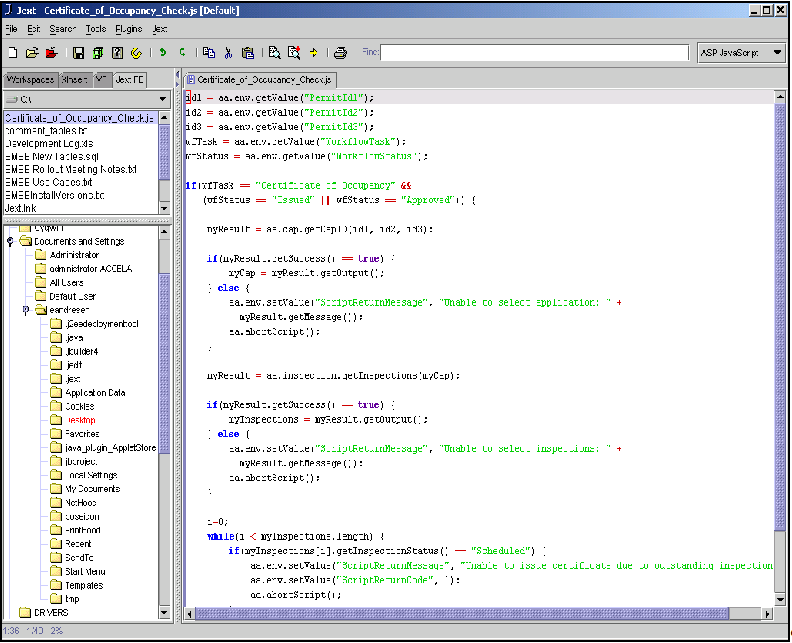
For Jext to recognize your scripts as JavaScript files you must save them with the extension “.js”. When opened in Jext, the editor highlights the script text in different colors to make it easier to read. Other useful features include a counter in the bottom left that tells you what line of the script that you are on, and a file explorer in the along the left side to make finding and opening files easier.